Python Change Variable and Loop Again

Welcome! If yous want to learn how to work with while loops in Python, then this article is for you.
While loops are very powerful programming structures that you can use in your programs to repeat a sequence of statements.
In this article, you will learn:
- What while loops are.
- What they are used for.
- When they should be used.
- How they work behind the scenes.
- How to write a while loop in Python.
- What infinite loops are and how to interrupt them.
- What
while Truthful
is used for and its general syntax. - How to use a
interruption
statement to cease a while loop.
You will larn how while loops piece of work backside the scenes with examples, tables, and diagrams.
Are yous ready? Allow'southward begin. 🔅
🔹 Purpose and Utilise Cases for While Loops
Allow'south start with the purpose of while loops. What are they used for?
They are used to repeat a sequence of statements an unknown number of times. This type of loop runs while a given condition is True
and it only stops when the status becomes False
.
When we write a while loop, we don't explicitly define how many iterations will be completed, nosotros just write the status that has to be True
to continue the procedure and False
to end it.
💡 Tip: if the while loop condition never evaluates to Faux
, then we will have an infinite loop, which is a loop that never stops (in theory) without external intervention.
These are some examples of real use cases of while loops:
- User Input: When we ask for user input, we demand to check if the value entered is valid. We can't possibly know in advance how many times the user volition enter an invalid input earlier the program can keep. Therefore, a while loop would be perfect for this scenario.
- Search: searching for an element in a data structure is another perfect utilize instance for a while loop because nosotros tin't know in advance how many iterations volition be needed to discover the target value. For case, the Binary Search algorithm tin can be implemented using a while loop.
- Games: In a game, a while loop could exist used to proceed the main logic of the game running until the player loses or the game ends. Nosotros can't know in advance when this will happen, then this is some other perfect scenario for a while loop.
🔸 How While Loops Work
Now that y'all know what while loops are used for, permit's meet their main logic and how they piece of work behind the scenes. Here we take a diagram:

Let's break this downwards in more detail:
- The process starts when a while loop is found during the execution of the program.
- The condition is evaluated to cheque if it'south
True
orFalse
. - If the status is
True
, the statements that belong to the loop are executed. - The while loop condition is checked once more.
- If the condition evaluates to
True
again, the sequence of statements runs again and the process is repeated. - When the condition evaluates to
False
, the loop stops and the program continues across the loop.
Ane of the most important characteristics of while loops is that the variables used in the loop condition are not updated automatically. We have to update their values explicitly with our code to make sure that the loop will eventually stop when the status evaluates to False
.
🔹 General Syntax of While Loops
Dandy. Now you lot know how while loops piece of work, so let'southward swoop into the code and encounter how you lot can write a while loop in Python. This is the basic syntax:

These are the main elements (in society):
- The
while
keyword (followed past a space). - A condition to determine if the loop will continue running or non based on its truth value (
True
orFalse
). - A colon (
:
) at the end of the outset line. - The sequence of statements that will be repeated. This block of lawmaking is chosen the "body" of the loop and it has to exist indented. If a statement is not indented, it volition non exist considered function of the loop (please see the diagram below).
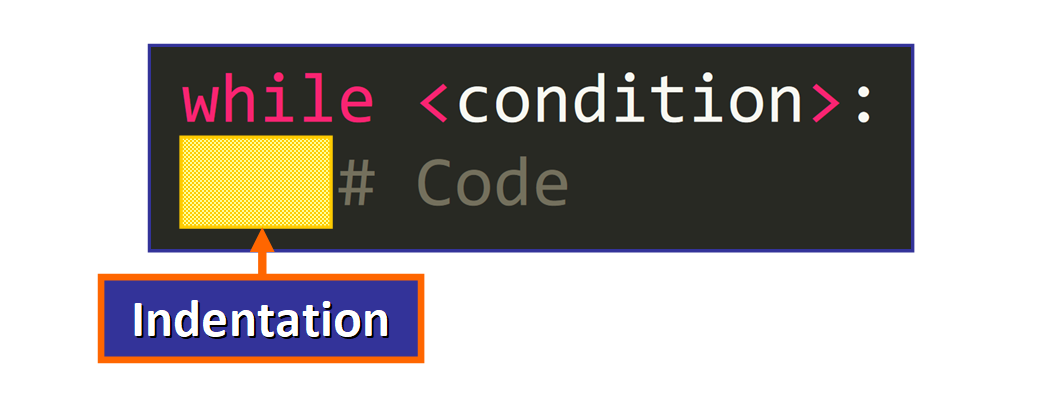
💡 Tip: The Python mode guide (PEP eight) recommends using four spaces per indentation level. Tabs should merely be used to remain consistent with code that is already indented with tabs.
🔸 Examples of While Loops
At present that you know how while loops work and how to write them in Python, let's see how they work behind the scenes with some examples.
How a Bones While Loop Works
Here nosotros accept a basic while loop that prints the value of i
while i
is less than 8 (i < eight
):
i = 4 while i < 8: print(i) i += 1
If we run the code, nosotros see this output:
4 5 6 7
Let's encounter what happens behind the scenes when the lawmaking runs:
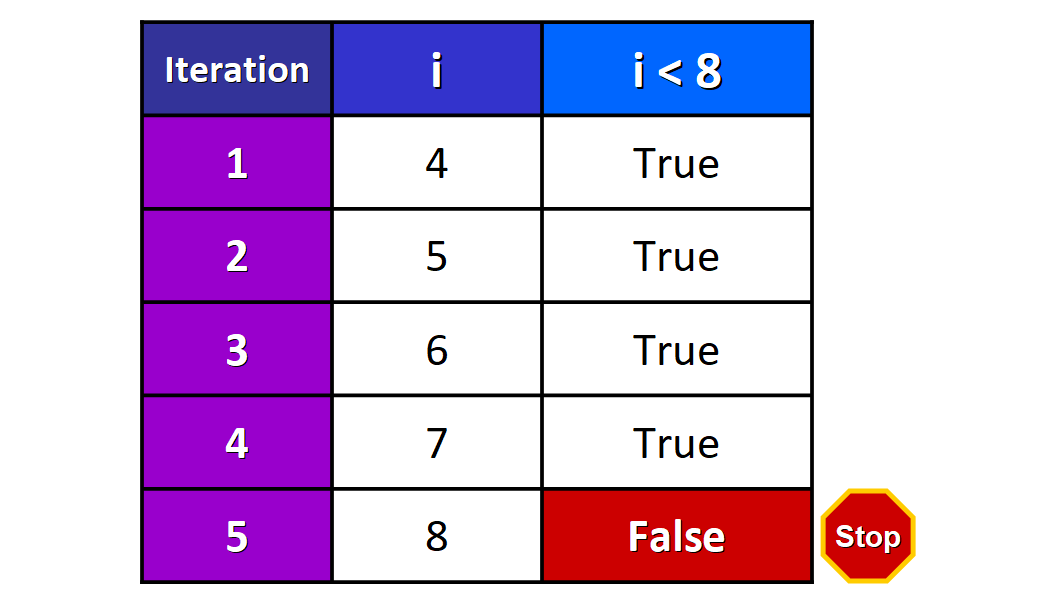
- Iteration i: initially, the value of
i
is iv, so the conditioni < eight
evaluates toTrue
and the loop starts to run. The value ofi
is printed (4) and this value is incremented by 1. The loop starts again. - Iteration 2: at present the value of
i
is 5, and then the conditioni < 8
evaluates toTruthful
. The body of the loop runs, the value ofi
is printed (five) and this valuei
is incremented by 1. The loop starts once more. - Iterations three and 4: The same procedure is repeated for the third and fourth iterations, so the integers 6 and vii are printed.
- Before starting the fifth iteration, the value of
i
iseight
. At present the while loop conditioni < 8
evaluates toFalse
and the loop stops immediately.
💡 Tip: If the while loop condition is Simulated
before starting the starting time iteration, the while loop will not even start running.
User Input Using a While Loop
Now let's meet an instance of a while loop in a programme that takes user input. We will the input()
part to enquire the user to enter an integer and that integer volition only exist appended to list if it'southward even.
This is the lawmaking:
# Ascertain the listing nums = [] # The loop will run while the length of the # list nums is less than 4 while len(nums) < four: # Ask for user input and shop information technology in a variable as an integer. user_input = int(input("Enter an integer: ")) # If the input is an fifty-fifty number, add it to the list if user_input % 2 == 0: nums.append(user_input)
The loop status is len(nums) < 4
, so the loop will run while the length of the listing nums
is strictly less than 4.
Allow'southward analyze this plan line past line:
- We offset by defining an empty list and assigning it to a variable called
nums
.
nums = []
- Then, we define a while loop that will run while
len(nums) < 4
.
while len(nums) < four:
- We ask for user input with the
input()
function and store it in theuser_input
variable.
user_input = int(input("Enter an integer: "))
💡 Tip: We need to convert (cast) the value entered past the user to an integer using the int()
part before assigning it to the variable considering the input()
function returns a string (source).
- Nosotros check if this value is even or odd.
if user_input % two == 0:
- If it's even, we suspend it to the
nums
list.
nums.append(user_input)
- Else, if information technology's odd, the loop starts again and the status is checked to determine if the loop should continue or not.
If we run this code with custom user input, nosotros get the post-obit output:
Enter an integer: three Enter an integer: 4 Enter an integer: 2 Enter an integer: 1 Enter an integer: seven Enter an integer: 6 Enter an integer: 3 Enter an integer: 4
This table summarizes what happens behind the scenes when the code runs:

💡 Tip: The initial value of len(nums)
is 0
because the list is initially empty. The last column of the tabular array shows the length of the listing at the end of the electric current iteration. This value is used to check the condition earlier the next iteration starts.
As yous tin can encounter in the tabular array, the user enters fifty-fifty integers in the second, third, 6th, and 8 iterations and these values are appended to the nums
list.
Earlier a "ninth" iteration starts, the status is checked once again but now it evaluates to Simulated
because the nums
list has four elements (length four), so the loop stops.
If we check the value of the nums
list when the process has been completed, we run across this:
>>> nums [4, 2, 6, iv]
Exactly what nosotros expected, the while loop stopped when the status len(nums) < 4
evaluated to Simulated
.
Now you know how while loops piece of work backside the scenes and you've seen some applied examples, so let's dive into a cardinal element of while loops: the condition.
🔹 Tips for the Condition in While Loops
Before you lot start working with while loops, you should know that the loop status plays a cardinal part in the functionality and output of a while loop.

You lot must be very careful with the comparison operator that you choose considering this is a very common source of bugs.
For example, common errors include:
- Using
<
(less than) instead of<=
(less than or equal to) (or vice versa). - Using
>
(greater than) instead of>=
(greater than or equal to) (or vice versa).
This can affect the number of iterations of the loop and even its output.
Let'due south see an instance:
If we write this while loop with the condition i < 9
:
i = 6 while i < 9: print(i) i += ane
Nosotros see this output when the code runs:
vi 7 8
The loop completes iii iterations and it stops when i
is equal to ix
.
This table illustrates what happens behind the scenes when the code runs:
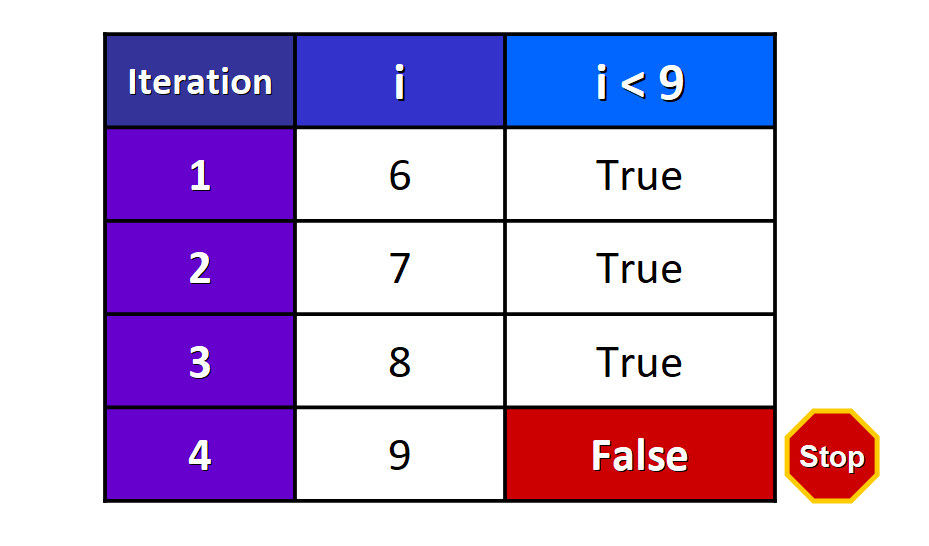
- Before the first iteration of the loop, the value of
i
is 6, so the statusi < 9
isTrue
and the loop starts running. The value ofi
is printed and then it is incremented by 1. - In the second iteration of the loop, the value of
i
is vii, so the statusi < 9
isTrue
. The body of the loop runs, the value ofi
is printed, and so it is incremented past 1. - In the third iteration of the loop, the value of
i
is 8, and so the conditioni < 9
isTrue
. The body of the loop runs, the value ofi
is printed, and then information technology is incremented by 1. - The condition is checked again before a 4th iteration starts, only now the value of
i
is ix, soi < ix
isFalse
and the loop stops.
In this case, nosotros used <
as the comparison operator in the condition, but what do you think will happen if we use <=
instead?
i = half-dozen while i <= 9: print(i) i += one
We run across this output:
6 7 viii 9
The loop completes one more than iteration because now we are using the "less than or equal to" operator <=
, so the condition is still True
when i
is equal to 9
.
This table illustrates what happens backside the scenes:

Four iterations are completed. The condition is checked again before starting a "fifth" iteration. At this point, the value of i
is 10
, and so the condition i <= 9
is Imitation
and the loop stops.
🔸 Space While Loops
Now you know how while loops work, but what exercise you lot think will happen if the while loop status never evaluates to Faux
?
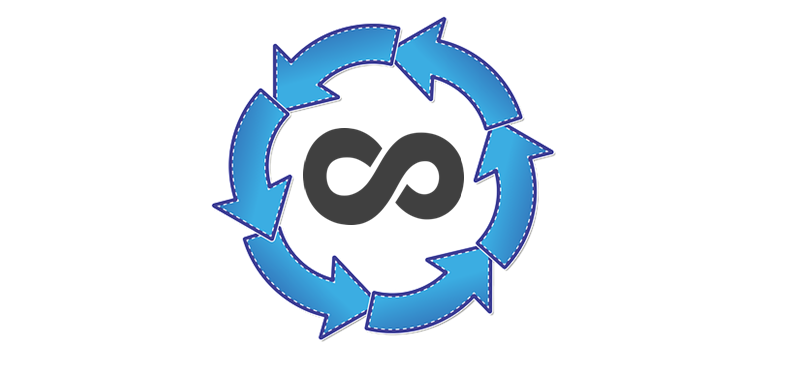
What are Space While Loops?
Remember that while loops don't update variables automatically (we are in charge of doing that explicitly with our code). Then there is no guarantee that the loop volition stop unless nosotros write the necessary code to make the condition False
at some signal during the execution of the loop.
If we don't practice this and the condition always evaluates to Truthful
, then we will have an space loop, which is a while loop that runs indefinitely (in theory).
Infinite loops are typically the consequence of a bug, just they can as well be caused intentionally when we want to repeat a sequence of statements indefinitely until a intermission
argument is found.
Let's see these 2 types of space loops in the examples below.
💡 Tip: A issues is an mistake in the program that causes incorrect or unexpected results.
Example of Space Loop
This is an example of an unintentional infinite loop acquired by a bug in the program:
# Define a variable i = 5 # Run this loop while i is less than 15 while i < xv: # Print a message print("Hullo, World!")
Analyze this code for a moment.
Don't you notice something missing in the torso of the loop?
That's right!
The value of the variable i
is never updated (it'due south always 5
). Therefore, the condition i < 15
is ever True
and the loop never stops.
If we run this code, the output volition exist an "space" sequence of Hullo, Globe!
letters because the body of the loop print("Hi, World!")
will run indefinitely.
Howdy, World! Hello, World! Howdy, World! Hello, World! Hullo, Globe! Hi, World! Hello, World! Hello, Globe! Hello, Earth! Howdy, World! Hullo, World! Hi, World! Hello, World! How-do-you-do, World! Hello, World! Hello, World! Hello, World! Hello, Earth! . . . # Continues indefinitely
To stop the program, we will demand to interrupt the loop manually by pressing CTRL + C
.
When we do, we will encounter a KeyboardInterrupt
error similar to this 1:

To set up this loop, nosotros will need to update the value of i
in the trunk of the loop to make certain that the condition i < 15
will eventually evaluate to False
.
This is one possible solution, incrementing the value of i
past 2 on every iteration:
i = v while i < 15: print("Hello, Earth!") # Update the value of i i += ii
Great. Now you know how to set space loops acquired by a bug. You just need to write code to guarantee that the condition will eventually evaluate to False
.
Let'south commencement diving into intentional infinite loops and how they piece of work.
🔹 How to Brand an Infinite Loop with While True
We can generate an infinite loop intentionally using while Truthful
. In this case, the loop will run indefinitely until the procedure is stopped past external intervention (CTRL + C
) or when a break
statement is found (yous will acquire more about intermission
in just a moment).
This is the basic syntax:

Instead of writing a condition subsequently the while
keyword, we just write the truth value directly to indicate that the status will always be True
.
Hither we accept an instance:
>>> while True: print(0) 0 0 0 0 0 0 0 0 0 0 0 0 0 Traceback (almost recent call concluding): File "<pyshell#2>", line 2, in <module> print(0) KeyboardInterrupt
The loop runs until CTRL + C
is pressed, but Python too has a pause
statement that we can use directly in our lawmaking to cease this blazon of loop.
The pause
statement
This argument is used to stop a loop immediately. You should think of it as a red "stop sign" that you can apply in your lawmaking to have more command over the beliefs of the loop.

According to the Python Documentation:
Thebreak
argument, similar in C, breaks out of the innermost enclosingfor
orwhile
loop.
This diagram illustrates the basic logic of the break
statement:

break
statement This is the basic logic of the break
statement:
- The while loop starts only if the status evaluates to
True
. - If a
interruption
argument is found at any signal during the execution of the loop, the loop stops immediately. - Else, if
pause
is not found, the loop continues its normal execution and information technology stops when the condition evaluates toFaux
.
We tin can use break
to stop a while loop when a condition is met at a item indicate of its execution, so you will typically observe it within a conditional statement, like this:
while True: # Code if <status>: break # Lawmaking
This stops the loop immediately if the condition is Truthful
.
💡 Tip: You tin can (in theory) write a break
statement anywhere in the body of the loop. It doesn't necessarily have to be part of a conditional, simply we ordinarily employ it to stop the loop when a given condition is True
.
Here nosotros take an case of break
in a while True
loop:
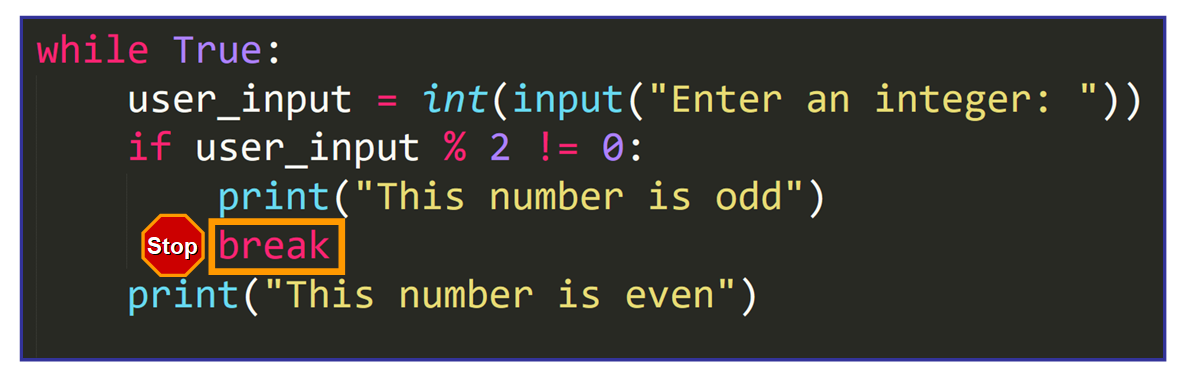
Let'due south see it in more detail:
The offset line defines a while True
loop that will run indefinitely until a break
statement is plant (or until it is interrupted with CTRL + C
).
while Truthful:
The second line asks for user input. This input is converted to an integer and assigned to the variable user_input
.
user_input = int(input("Enter an integer: "))
The third line checks if the input is odd.
if user_input % ii != 0:
If it is, the message This number is odd
is printed and the break
statement stops the loop immediately.
print("This number of odd") break
Else, if the input is even , the message This number is even
is printed and the loop starts again.
print("This number is even")
The loop will run indefinitely until an odd integer is entered because that is the just way in which the break
argument will exist found.
Here nosotros take an example with custom user input:
Enter an integer: 4 This number is even Enter an integer: half-dozen This number is even Enter an integer: viii This number is even Enter an integer: three This number is odd >>>
🔸 In Summary
- While loops are programming structures used to echo a sequence of statements while a condition is
True
. They terminate when the condition evaluates toFalse
. - When you write a while loop, y'all demand to make the necessary updates in your code to brand sure that the loop will somewhen finish.
- An infinite loop is a loop that runs indefinitely and information technology only stops with external intervention or when a
break
argument is found. - You tin cease an infinite loop with
CTRL + C
. - You tin can generate an infinite loop intentionally with
while Truthful
. - The
suspension
statement can be used to stop a while loop immediately.
I really hope you liked my commodity and establish it helpful. At present yous know how to work with While Loops in Python.
Follow me on Twitter @EstefaniaCassN and if you lot desire to larn more well-nigh this topic, check out my online course Python Loops and Looping Techniques: Beginner to Avant-garde.
Learn to code for gratuitous. freeCodeCamp'southward open source curriculum has helped more than than 40,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-while-loop-tutorial/
0 Response to "Python Change Variable and Loop Again"
Post a Comment